Showing current Kubernetes cluster in Powershell prompt
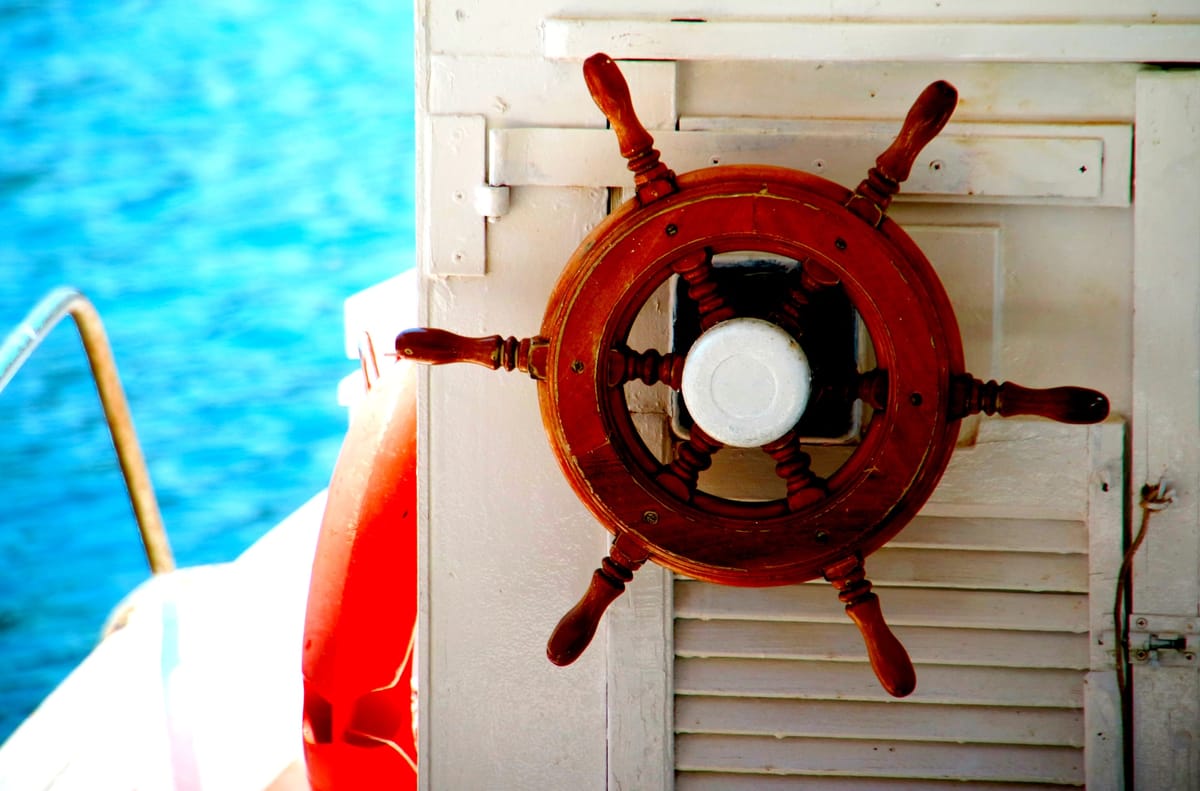
After nearly clearing the wrong Kubernetes cluster, I decided to add the name of the currently active cluster to my Powershell prompt. There are plenty of plugins to do this in Bash/Zsh/fish, but not as many for Powershell.
It's not hard to do, but the syntax and tools you use are definitely different from Unix tools.
First, let's get the name of the currently active cluster. We can look through the ~/.kube/config
file for the field called current-context
. On Linux, I would use grep
to extract this, on Windows we use Select-String
, which receives a regex to match and outputs the matches, as objects. We look for the first match and for the second group (which would be the first and only capture group in our regex) and we put it's value in $ctx
variable. This should output the current cluster name.
$K8sContext=$(Get-Content ~/.kube/config | Select-String -Pattern "current-context: (.*)")
$ctx=$K8sContext.Matches[0].Groups[1].Value
Write-Host $ctx
And now, to edit the prompt, you must modify your PS1 profile. If you don't have one, then create a file in C:\Users\<USERNAME>\Documents\WindowsPowerShell\profile.ps1
. If you already have a profile (which might be a global one or a per user one), edit it and add the following:
function _OLD_PROMPT {""}
copy-item function:prompt function:_OLD_PROMPT
function prompt {
$K8sContext=$(Get-Content ~/.kube/config | Select-String -Pattern "current-context: (.*)")
If ($K8sContext) {
$ctx=$K8sContext.Matches[0].Groups[1].Value
# Set the prompt to include the cluster name
Write-Host -NoNewline -ForegroundColor Yellow "[$ctx] "
}
_OLD_PROMPT
}
The prompt
function is called to write out the prompt. First we save a copy of the original prompt into the _OLD_PROMPT
variable and then we define our new prompt function. In the new function we do the above snippet, with an added check to make sure we add something to the prompt if there was a match for our regex. I put the name of the cluster in square brackets to make it visually distinct from the Python virtual environment name, which comes afterward in parenthesis.
The result is as follows:
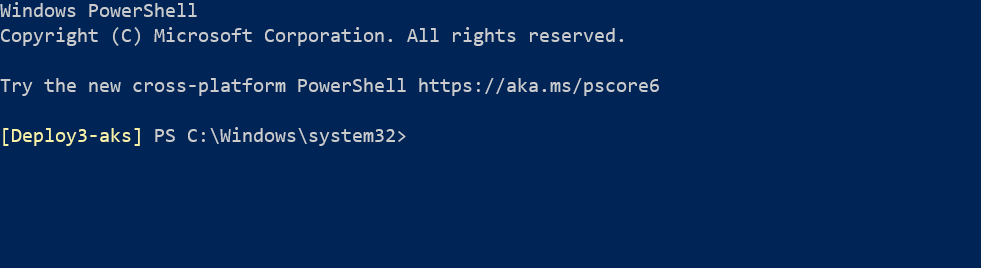
Good luck with not nuking the wrong Kubernetes cluster!
I’m publishing this as part of 100 Days To Offload - Day 29.