Making a loudness monitor for online meetings
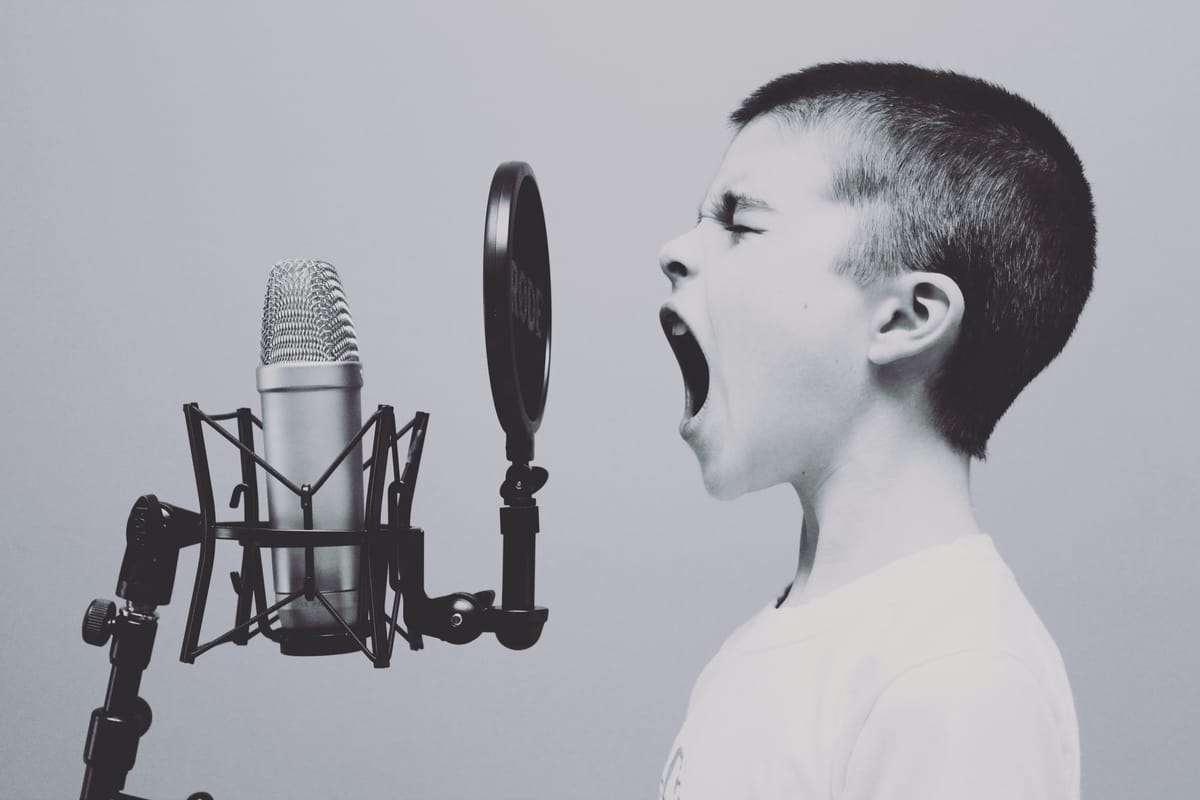
As I work from home 90% of the time, I run into a small issue during meetings: I sometimes speak too loudly. Before my daughter Gloria arrived, this was something that annoyed my wife and others in the house, but now, when Gloria is sleeping, this is not just an annoyance, it's a BIG problem, because nobody wants to wake up a toddler.
While I do have monitoring that alerts me via Signal that I'm speaking too loud (my wife), I wanted to write a program to do that all the time, in the spirit of using programming to make my life nicer.
So I started to look for a Python library that can give me information about the sound level from my microphone. A quick Kagi search revealed several options, but sounddevice
seemed like the best one.
The first step is to identify the microphone. For that I need the name as it's know to the operating system. I can get that by running the following code in a Python console:
> import sounddevice as sd
> sd.query_devices()
0 Microsoft Sound Mapper - Input, MME (2 in, 0 out)
1 Microphone (Yeti Stereo Microph, MME (2 in, 0 out)
2 Microphone (WO Mic Device), MME (2 in, 0 out)
....
> sd.query_devices()[1]['name']
'Microphone (Yeti Stereo Microph'
I get a long list of stuff, but I see something with Yeti in the name so I grab that one.
Now let's start listening to the microphone. Sounddevice offers a callback based API, where it passes along the raw audio data received from the microphone. From that, I estimate the loudness by calculating the norm of the sound:
import numpy as np
import sounddevice as sd
def print_sound(indata, frames, t, status):
volume = np.linalg.norm(indata) * 10
print(volume)
name = 'Microphone (Yeti Stereo Microph'
with sd.InputStream(device=name,callback=print_sound):
for i in range(5):
sd.sleep(1000)
Running this gives something as follows. Can you guess where I snapped my fingers?
0.3724626451730728
0.6015866994857788
0.9348087012767792
0.7427176833152771
0.8615989238023758
0.7162655889987946
0.5638395622372627
0.7117109000682831
59.17434215545654
50.70761203765869
20.951063632965088
14.069621562957764
9.29598331451416
5.908793210983276
3.782018721103668
2.402055263519287
1.7902085185050964
1.1522774398326874
0.793280228972435
The next step is to make it warn me when I speak too loud. For this I keep a buffer of the latest sound intensities in order to be able to detect when either something loud has been happening for a long time or if a really loud noise happened in the last frames:
import time
from collections import deque
import numpy as np
import sounddevice as sd
last_alert = time.time() - 10
q = deque(maxlen=200)
def print_sound(indata, frames, t, status):
global last_alert
volume_norm = np.linalg.norm(indata) * 10
q.append(volume_norm)
last_elements = [q[i] for i in range(-min(50, len(q)), 0)]
recent_avg_sound = sum(last_elements) / len(last_elements)
num_high_count = len([x for x in q if x > 20])
if num_high_count > 30 or recent_avg_sound > 50:
if time.time() - last_alert > 10:
print(f"You are speaking at {volume_norm:.2f}. Think of Gloria!\a")
last_alert = time.time()
name = 'Microphone (Yeti Stereo Microph'
with sd.InputStream(device=name,callback=print_sound):
while True:
sd.sleep(1000)
Now, when running from a Terminal (either on Windows or Linux), this will make a bell sound if in the last 5 seconds (that's about 200 frames) there have been more than 30 frames with loudness over 20 or if in the last second the average was over 50 (this would mean a really loud sound).
If you want to run this outside of Terminal, you can use beepy
for example to make sounds and replace the print
statement with this:
from beepy import beep
beep(sound="error")
To run this on startup on Windows, I created the following ps1 script in the startup folder:
C:\Users\Roland\Programming\mic_check\.venv\Scripts\pythonw.exe C:\Users\Roland\Programming\mic_check\mic_check.py
Another improvement would be to make it easier to see current loudness and to be able to quit it easily (because the ps1 script runs in the background). For this I used the infi.systray
library on Windows:
from infi.systray import SysTrayIcon
def quit(systray):
global still_on
still_on = False
menu_options = ()
systray = SysTrayIcon("icon.ico", "Mic check tray icon", menu_options, on_quit=quit)
systray.start()
still_on = True
name = 'Microphone (Yeti Stereo Microph'
with sd.InputStream(device=name,callback=print_sound):
while still_on:
sd.sleep(1000)
And now, hopefully I'll learn to control my loudness better!
You can find the full code here.